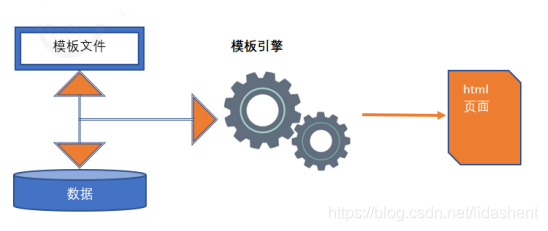
SpringBoot Thymeleaf 模板引擎
一、介绍
SpringBoot主要支持Thymeleaf、Freemarker、Mustache、 Groovy Templates等模板引擎。Thymeleaf可以轻易地与SpringMVC等Web框架进行集成。Thymeleaf语法并不会破坏文档的结构,所以Thymeleaf模板依然是有效的HTML文档。模板还可以被用作工作原型,Thymeleaf会在运行期内替换掉静态值。它的模板文件能直接在浏览器中打开并正确显示页面,而不需要启动整个Web应用程序。
二、配置
引入依赖
<!-- 引入Thymeleaf模板引擎 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
二、模板
在 resources 包下创建 templates.index.html,一下为一个简单的模板
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>用户信息</title>
<meta name="author" >
</head>
<body>
<a class="url" th:href="@{${url}}" target="_blank">点击下载App</a>
</body>
</html>
在yml文件中配置:
spring:
thymeleaf:
mode: HTML5
encoding: UTF-8
cache: false #使用Thymeleaf模板引擎,关闭缓存
servlet:
content-type: text/html
三、业务逻辑层
// 使用 FileOutputStream 若路径中没有该文件会自动创建, 没有该文件夹就会报 (系统找不到该路径);
String path = "./" + System.currentTimeMillis() + ".html";
File file = new File(path);
Context context = new Context();
// 往html中添加内容
// url = APP 下载链接,用来替换到模板中'url'
context.setVariable("url", urlApp);
// index.html 为模板的名称,执行 TemplateUtils.process 方法后会在指定(file)路径生成模板文件。
boolean process = TemplateUtils.process("index.html", context, file);
// 上传OSS 返回 URL
// ....
// 删除 生成路径的文件
cn.hutool.core.io.FileUtil.del(file);
// 拿到 URL 可以做 后面的逻辑
// ...
工具方法:
@Resource
public static final TemplateEngine ENGINE = ApplicationContextHolder.getBean(TemplateEngine.class);
/**
* 生成静态文件
*
* @param freeTempName 模板名称
* @param context 数据内容
* @param outFilePath 输出路径
* @return
*/
public static boolean process(String freeTempName, Context context, File outFilePath) {
FileWriter fileWriter = null;
try {
fileWriter = new FileWriter(outFilePath);
ENGINE.process(freeTempName, context, fileWriter);
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
} finally {
try {
if (fileWriter != null) {
fileWriter.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Component
public class ApplicationContextHolder implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext ctx) throws BeansException {
applicationContext = ctx;
}
public static ApplicationContext getApplicationContext() {
return applicationContext;
}
public static <T> T getBean(Class<T> clazz) {
return applicationContext.getBean(clazz);
}
@SuppressWarnings("unchecked")
public static <T> T getBean(String name) {
return (T) applicationContext.getBean(name);
}
}
常见属性:SpringBoot——Thymeleaf常见属性-使用th:each遍历数组、List、Map_thymeleaf遍历数组
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果