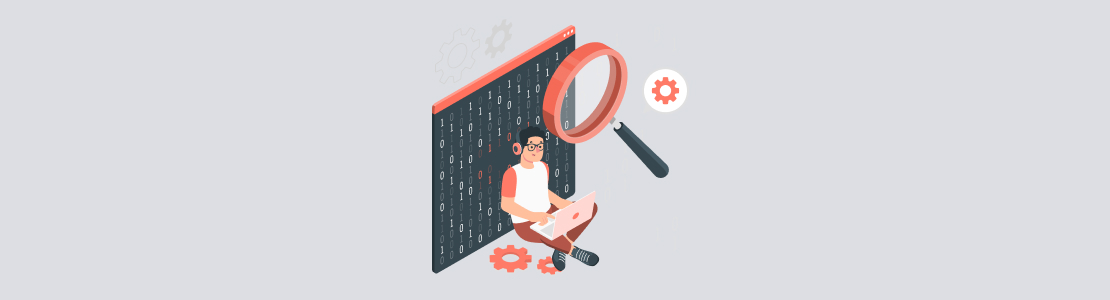
Java 动态代理,动态的执行Mapper中的某个方法
Java 动态代理,动态的执行Mapper中的某个方法
1、 创建动态代理类
/**
* @ClassName: MyInvocationHandler
* @author: anqin
* @Description: 动态代理类
* @date: 2022/12/3 2:30
*/
public class MyInvocationHandler implements InvocationHandler {
/**
* 目标对象的Mapper
*/
private Object target;
public MyInvocationHandler(Object target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
return method.invoke(target, args);
}
}
2、先通过动态代理获取该类,在执行指定的方法
/**
* 注入所需要的 SqlSession
*/
@Resource
private org.apache.ibatis.session.SqlSession sqlSession;
/**
* 如根据以下:在 UserMapper 中 需要动态的 根据 用户 id 查询该用户
*
* @param clazz 指定 UserMapper 字节码文件
* @param methodName 根据 用户 id 查询用户的 方法名称 如 'queryUserById'
* @param methodParam 该 ‘queryUserById’ 方法中所需要的参数(可以为多个)
* @return 返回的结果
*/
public Object dynamicProxy(Class<?> clazz, String methodName, String methodParam) {
try {
// 获取该类
Object mapper = Proxy.newProxyInstance(clazz.getClassLoader(),
new Class[]{clazz},
new MyInvocationHandler(sqlSession.getMapper(clazz)));
// 获取该类中 指定的方法
// 参数一:类名称
// 参数二(多参):该指方法的参数类型 字节码
Method method = clazz.getMethod(methodName, String.class);
// 执行获取的方法
// 参数一:动态代理获取的结果
// 参数二(多参):需要执行的参数结果,如:id 为 1001
return method.invoke(mapper, methodParam);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
3、测试
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class ProxyTest {
@Test
public void test() {
CustomizeTableShardingAlgorithm sharding = new CustomizeTableShardingAlgorithm();
int proxy = (int) sharding.dynamicProxy(CommonMapper.class, "isTable", "hmp_user");
System.out.println(proxy);
}
}
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果